Web Scraping: Best Methods and Practices
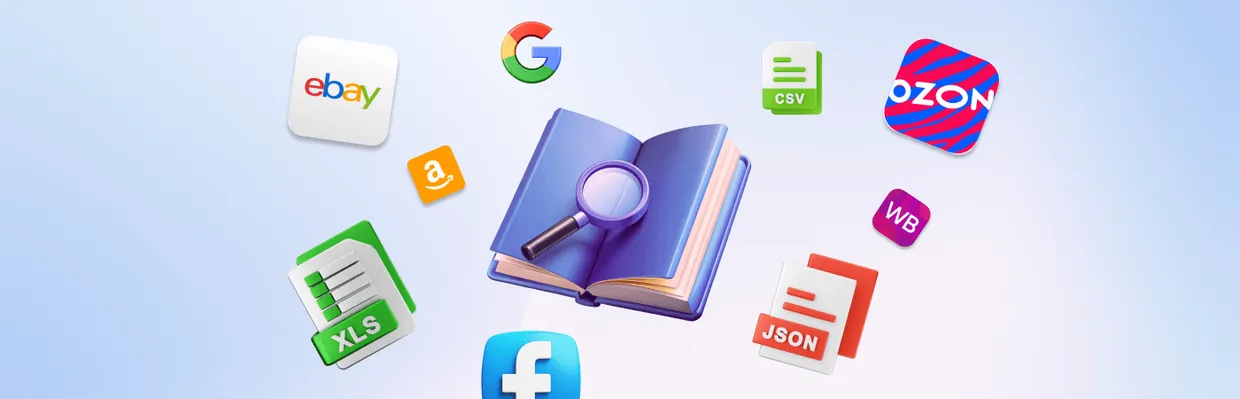
Hi! Web scraping has become an integral part of modern business. So, in this article, I will share the leading methods and practices of web scraping, talk about its challenges, and how to overcome them.
What is Web Scraping?
Web scraping is an automated process of collecting data from web pages. In other words, it's a process where a program sends requests to a website, extracts the HTML code, and analyzes it to pull out the necessary information. This approach is particularly useful when you need to gather large amounts of data in a short period. For example, if you need to collect prices from several marketplaces or analyze comments from forums.
Why Do You Need Web Scraping?
Imagine you need to collect data from thousands of pages manually. It would take an enormous amount of time and effort. Web scraping automates this process. It allows you to:
- Analyze data easily. For instance, if you're working with marketplaces, scraping helps track price changes, product availability, and evaluate competitors.
- Study trends. Web scraping allows you to extract data from news sites, social media, and forums to analyze user preferences and behavior.
- Stay updated with the latest changes. In some cases, website data changes, and scraping helps quickly obtain updated information, be it product availability or price updates.
- Create the most effective strategies. By analyzing massive datasets, you can plan and create successful marketing strategies, considering both positive and negative experiences of competitors, which can help your business become more successful.
Let me give you an example of how scraping can be applied in real life. For instance, to identify the most popular topics and successful audience engagement strategies, an SMM specialist sets up a scraper to collect data on comments and likes on competitors' posts. And there are plenty of such examples; scraping is one of the foundations of a successful business these days. But it's not all smooth sailing.
How Do Websites Detect Scrapers?
Many website owners are against scraping, even though the information on their sites is publicly available. They actively take measures to prevent automated data collection. I've listed the most common methods of protecting against data extraction from websites below:
Rate Limiting
Many websites use rate limiting to protect against automated scrapers. This method sets limits on the number of requests that can be sent from a single IP address within a short period. It helps not only prevent server overload but also restricts bot activity. Some websites are configured to block only certain actions if the limit is exceeded (e.g., creating accounts or submitting forms), while other actions remain available, making it harder to detect the block. To bypass these restrictions, use rotating proxies. This allows you to change your IP address with each new request, avoiding being blocked.
CAPTCHA
CAPTCHA is one of the most effective tools to protect websites from scraping. It activates during suspicious activity related to a large number of requests or unnatural user behavior. Modern CAPTCHAs, such as Google reCAPTCHA, analyze user behavior on the page, while Invisible CAPTCHA can trigger without user intervention if a bot leaves suspicious digital fingerprints. To bypass such systems, a good anti-detect browser is needed, which alters the browser fingerprint, mimics real user behavior, and uses high-quality proxies. Sometimes anti-detect browsers can work with CAPTCHA solvers to automatically bypass the verification.
IP Blocks
IP address blocking usually occurs when too many requests are made in a short period, and the website perceives this as suspicious behavior, blocking them. It's worth noting that websites can block both individual IPs and entire ranges, especially if these IP addresses belong to large cloud providers like AWS or Google Cloud. To most effectively bypass this type of protection, I would recommend using rotating mobile or residential proxies, as they are harder to detect and block.
Website Structure Changes
Some websites regularly change their HTML markup, which complicates the work of scrapers. The site can remove or change CSS class names, restructure the DOM (Document Object Model), and add dynamic CSS classes that change with each new request. These changes are especially common on websites that use JavaScript for dynamic content loading. To scrape websites with such protection methods, it is necessary to regularly update scripts and check the relevance of the HTML structure.
JavaScript-heavy Web-Sites
Many modern websites rely on JavaScript to load content, making scraping more difficult. Simple HTML extraction will no longer provide the necessary data, as most content loads only after the page has fully loaded. To work around this problem, headless browsers, often used with libraries like Puppeteer or Selenium, are employed. These libraries allow the page to be fully rendered like in a regular browser, retrieving all the information at once. Moreover, websites can hide API requests or protect them with additional authentication, adding more complexity.
Slow Page Load
When a large number of requests are made to a site or when the server is under heavy load, pages may load slowly, making it difficult for scrapers to work. Some sites intentionally slow down the response speed if they detect abnormal activity, forcing the scraper to stop working due to timeouts. To avoid this, you can use the retry request feature and avoid exceeding request speed limits.
Stages of the Web Scraping Process
Let’s now look at the key stages of scraping:
- Collecting the page's source code. In other words, it's sending an HTTP request to the server to get the HTML code of the page. This process is similar to how a browser works when loading a site, but instead of rendering the page visually, you receive its source code. In Python, the "Requests" library is perfect for this task, allowing you to easily send GET requests and get the content of the page.
- Extracting the needed data. After obtaining the HTML code, we use a parser, such as Beautiful Soup. This is one of the popular libraries for Python, which helps parse the HTML code, find the necessary elements (e.g., headers, tables, or links), and extract data from them. At this stage, it's essential to carefully analyze the page structure to properly configure the parser to find the required elements and data.
- Formatting and saving the data. Next, the data needs to be converted into a convenient format, whether it be CSV, JSON, or any other database that suits your needs. At this stage, it's important to organize the data properly so that it is easily accessible and can be used in the future for analysis or processing.
Methods of Web Scraping
In this section, we’ll discuss two methods of scraping, for beginners who are just studying this topic and for advanced users.
Simple Scraping
If you are just starting with scraping, don't want or can't write a code, you can use ready-made tools available online. There are many such tools, for example, Octoparse or ParseHub, which offer visual interfaces for creating scraping scripts. These apps make scraping accessible even for those who don't understand programming.
Octoparse – A program with a graphical interface that allows you to easily collect data. It supports data collection from both simple and dynamic sites.
ParseHub – Another popular service with the ability to scrape pages where content is loaded not immediately but during interaction with the site.
Programmatic Scraping
For more complex tasks or to have full control over the scraping process, it's better to use specialized libraries. These libraries can be used with programming languages like Python and JavaScript, allowing you to adapt the scraper to specific tasks and requirements.
Beautiful Soup (Python)
This library is designed for easy data extraction from HTML and XML documents. It is perfect for static pages where the content is available immediately after loading the HTML. Beautiful Soup makes scraping simple and efficient, especially for small projects or for parsing data that is fully presented on the site right after loading.
Code example:
import requests from bs4 import BeautifulSoup
Retrieve the content of the page response = requests.get("https: //example, com") soup = BeautifulSoup(response.text, 'html.parser')
Extract and print all h3 headers for headers in soup.find_all('h3'): print(headers.text)
Puppeteer (JavaScript)
This is a powerful tool for working with JavaScript-heavy sites. Puppeteer launches a Chrome browser in headless mode, which fully renders the page, including executing JavaScript, making it ideal for dynamic sites where data is loaded after rendering. Puppeteer allows automating complex scenarios of interaction with a web page, such as filling out forms, navigating pages, and taking screenshots. \
Code example:
const puppeteer = require('puppeteer');
(async () => {
Launching the browser in headless mode const browser = await puppeteer.launch(); const page = await browser.newPage();
Navigating to the page await page.goto('https: //example, com');
Extracting and printing the page title const title = await page.title(); console.log( Page title: ${titlee});
Closing the browser await browser.close(); })();
Types of Web Scraping
Web scraping can be divided into two main types: scraping static and dynamic pages. Depending on how the content is loaded on the webpage, the appropriate methods and tools for extracting data are chosen.
Scraping Static Pages
Static pages load all the content immediately upon loading the HTML code of the page. This means that the data is directly available in the HTML source and does not require additional processing, such as executing JavaScript. For scraping such pages, simple tools that work with HTML, like Beautiful Soup, are suitable.
The main advantages of scraping static pages are simplicity and speed. Since the HTML is fully loaded right away, the scraper can easily extract the necessary data without the need for additional scripts or browser simulation.
Examples of sites with simple static content:
- News sites – Many news portals provide articles in static HTML without dynamic data loading.
- Blogs – Personal or corporate blogs often use static pages to display content.
- Information portals – Sites containing reference information, documents, or other textual data often do not depend on JavaScript for loading content.
Scraping Dynamic Pages
Dynamic pages load the main HTML code right away, but part of the data appears only after the page has fully loaded. This makes scraping more difficult, as standard libraries like Beautiful Soup cannot interact with JavaScript. For such tasks, tools capable of rendering the page, interacting with it and executing scripts, as a real browser does, are needed. Examples of such tools include Puppeteer, Selenium, and Playwright, which run the browser in a so-called “headless” mode, simulating a real browser. The problem with dynamic pages is that many sites load data as the user interacts with the site. For example, new elements may appear when scrolling, and data may load through AJAX requests. In this case, regular tools cannot immediately get all the content since it loads after certain actions.
Examples of dynamic sites:
- Social networks – Platforms like Facebook, Instagram, or Twitter actively use JavaScript for dynamic content loading.
- Large e-commerce platforms – Online stores like Ozon, WB, Amazon, AliExpress, etc., load product and filter data dynamically via AJAX requests depending on user actions.
- User-generated content platforms – Sites like YouTube or Reddit load content (videos, comments, posts) based on user activity, using JavaScript.
Practical Tips for Successful Web Scraping
To make your scraping successful and not trigger a negative reaction from websites, it's essential to avoid creating excessive load on web resources. Let's now go over the key practical tips and recommendations for successful scraping.
1. Follow the site's rules
Every website has its own terms of use, which should be followed. Before you start scraping, make sure to check the robots.txt file. This file contains instructions for web crawlers on which pages can be scraped and which cannot. Although following these instructions is not mandatory from a technical point of view, non-compliance may lead to legal consequences or a block from the resource.
Also, don’t ignore the website's terms of service (ToS). On many websites, especially social networks and large platforms, scraping data behind a login (e.g., personal pages) can violate their rules and lead to legal questions.
2. Speed and load on the website
When collecting data from small sites or resources with limited bandwidth, try not to create excessive load by sending a bunch of HTTP requests. Add delays between requests (usually from a few seconds to a minute) and limit the number of requests within a certain period. This is especially important when working with small websites that can be easily overwhelmed by a large number of requests.
In such cases, I would recommend scraping during low-traffic times (e.g., at night) to minimize any negative consequences for the resource's operation.
3. Use APIs when possible
Some websites provide official APIs for accessing data. Using an API is not only an ethical way to get data but also a more efficient method. APIs often provide structured data and reduce the load on the server. If the website you're planning to scrape offers an API, it's better to use it rather than scraping the page directly.
4. IP Rotation
To prevent website blocks, it's important to use IP rotation. If too many requests are sent from a single IP address, the website may automatically block it as suspicious activity. Using anti-detect browsers along with proxies that allow IP rotation can help avoid this problem. It’s also worth noting that some websites actively block cloud IP addresses, so it's better to choose residential or mobile proxies.
5. Use Anti-detect Browsers
To mask automated scraping, especially when working with websites that actively use JavaScript and other dynamic elements, it's recommended to use anti-detect browsers. They help conceal the fact of automated data collection by changing browser fingerprints (user-agent, request headers, cookies, etc.), making your scraper less noticeable to the website’s defense systems.
6. Behave as a Real User
Websites can track user behavior, and if it seems suspicious (e.g., too fast actions or repetitive requests), they may block access. To avoid this, scrapers should mimic the behavior of a real user. This can include random delays between requests, using different user-agent (browser fingerprints), and simulating actions such as scrolling or clicking. In other words, do everything possible so that the system perceives the bot as an ordinary user.
7. Regularly Update Your Scraper
Websites constantly change their structure, add new elements, or modify existing ones. This can break your scraper if it isn't updated. To keep the scraper effective and stable, it's necessary to regularly check the website’s structure and make corresponding changes to its code. It’s also important to test it on different pages to prevent issues.
8. Act as Naturally as Possible
Not only should you mimic human behavior, but the overall rhythm of interaction with the site should be as natural as possible. Add random intervals between requests, and avoid creating repetitive patterns that can be easily tracked. For example, requests with identical time intervals or continuous requests for several hours can easily be flagged as suspicious.
Conclusion
Web scraping is a powerful tool for automating data collection, opening up vast opportunities for analysis and decision-making. However, successful scraping requires not only the right tools but also adherence to certain rules, such as IP rotation and using anti-detect browsers to bypass website protection. By following the methods described in this article, you'll be able to efficiently collect data, avoiding blocks and not violating website rules.

Undetectable - the perfect solution for